Using the Charge Stability Diagram Models
Installation
We recommend using pip, poetry, or uv to install the package.
Authentication
The SDK requires an API key for authentication. Sign in and create a new API key. Remember, your API key is your access secret—keep it safe with environment variables.
Using environment variables:
Python
Or provide the API key directly:
Python
Usage Examples
Using the Charge Stability Diagram Segmenter
The Charge Stability Diagram Segmenter is a model that can segment a given current measurement into different charge stability regimes: no dot (0), single dot (1), and double dot (2).
You can download an example file to follow along with the example:
Python
Output
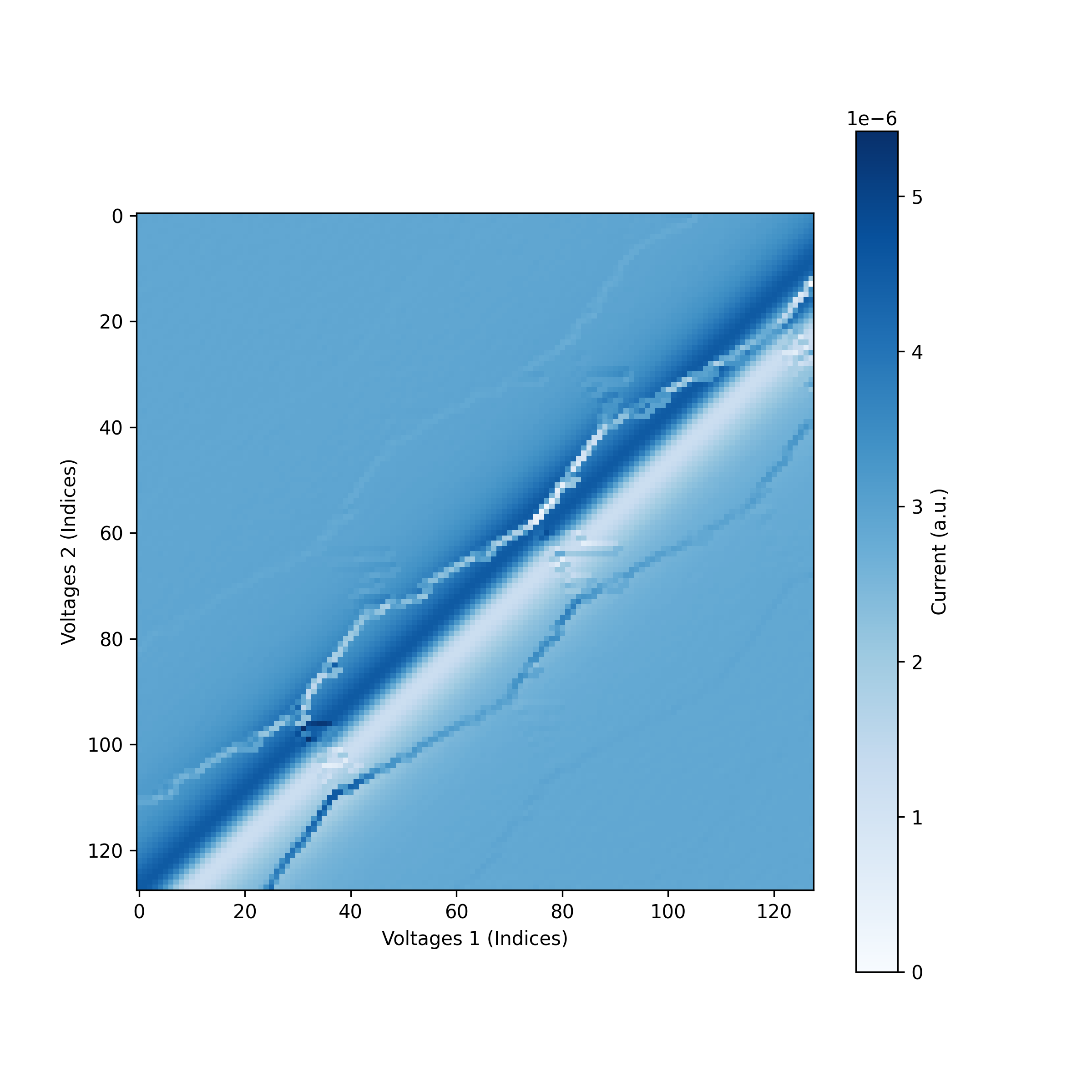
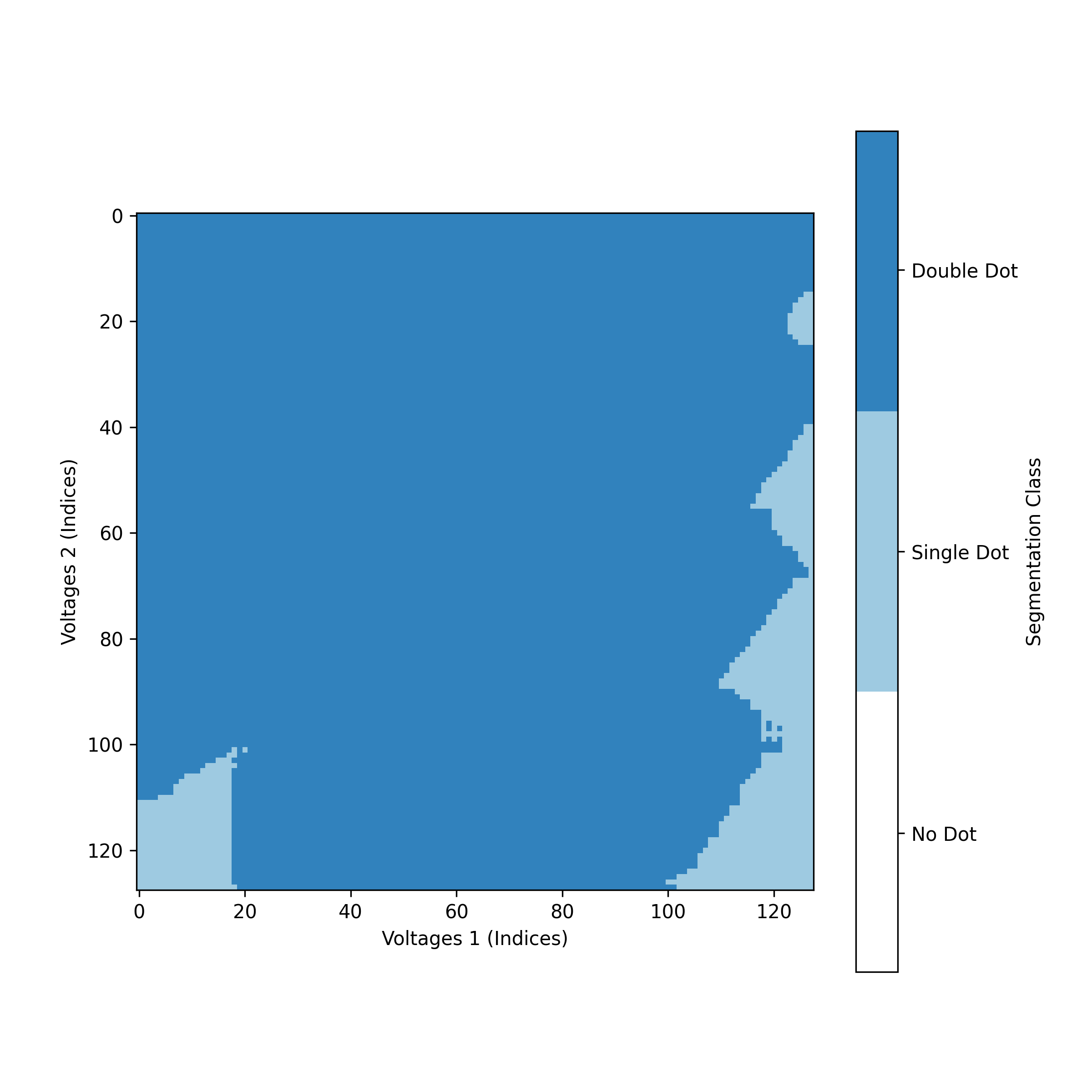
Data Requirements
Charge Stability Diagram Data
- Shape: (128, 128)
- 2D array of current values
Important Notes for Charge Stability Diagram Data
- For best results, input dimensions should be (128, 128) - you may need to interpolate/downsample your data.
- Each current value in the input array corresponds to a point in the charge stability diagram for a set of two plunger gate voltages.