Using the Turn-On Models
Installation
We recommend using pip, poetry, or uv to install the package.
Authentication
The SDK requires an API key for authentication. Sign in and create a new API key. Remember, your API key is your access secret—keep it safe with environment variables.
Using environment variables:
Python
Or provide the API key directly:
Python
Usage Examples
Using the Turn-On Classifier
The Turn-On Classifier is a model that can classify a given current measurement as either in the Turn-On regime or not.
You can download an example file to follow along with the example:
Python
Output
Using the Turn-On Parameter Extractor
The Turn-On Parameter Extractor is a model that can extract the parameters of a given current measurement.
You can download an example file to follow along with the example:
Python
Output
Plotting the Output
Python
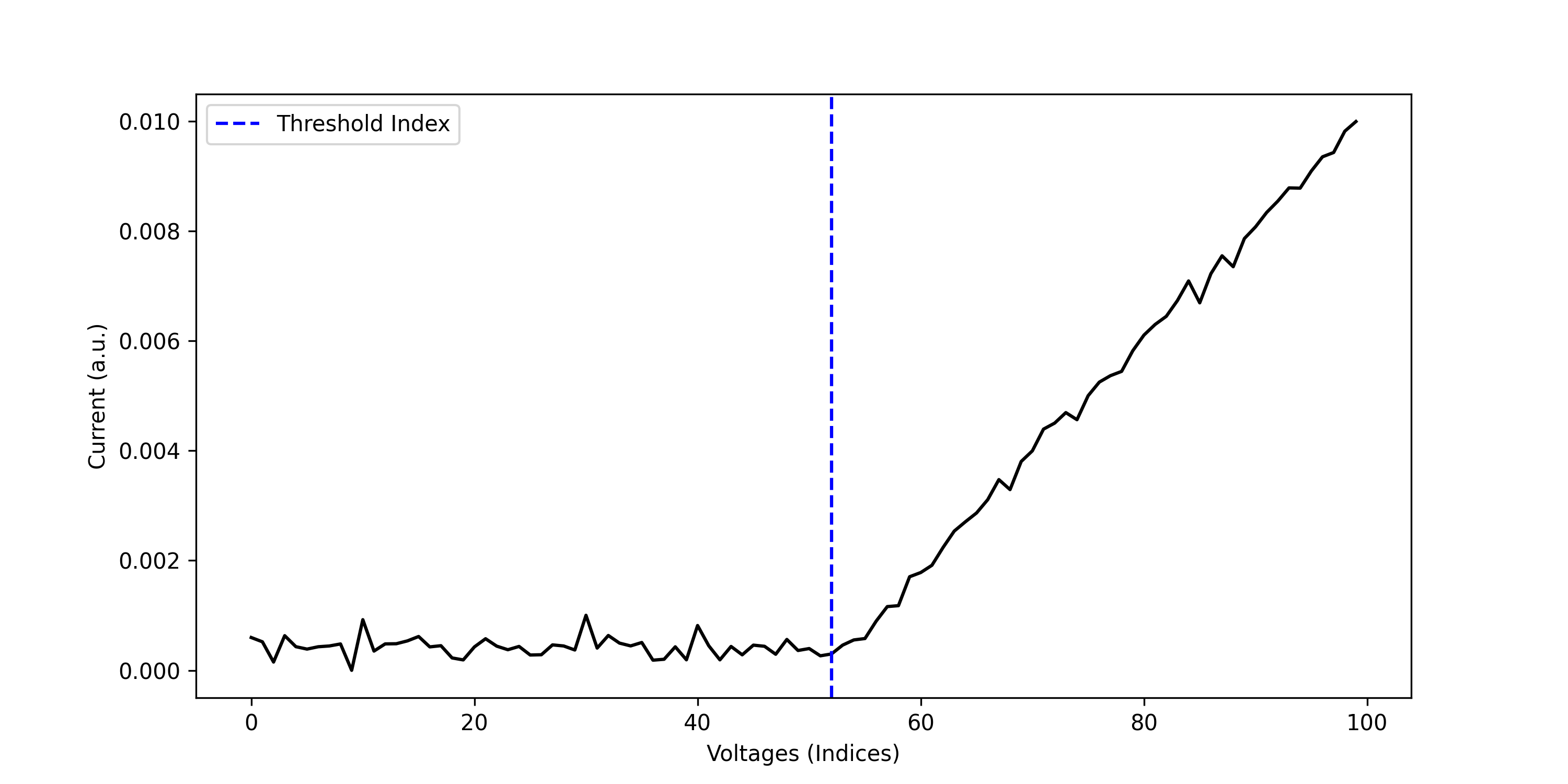
Data Requirements
One-dimensional Current Data
- Shape:
(n, )
- 1D array of current values
Important Notes for Voltage-Current Data
- The array must be of shape (n, ) where n is the number of current values in the measurement.
- Analysis is outputted in terms of indices of the input array.
- The models automatically handle scaling and normalization internally.
- For best results, ensure your data has sufficient resolution in regions of interest.